Blog
Understanding the Concept of Possiblyethereal: A Journey into the Unseen
Published
1 month agoon
By
Ali baba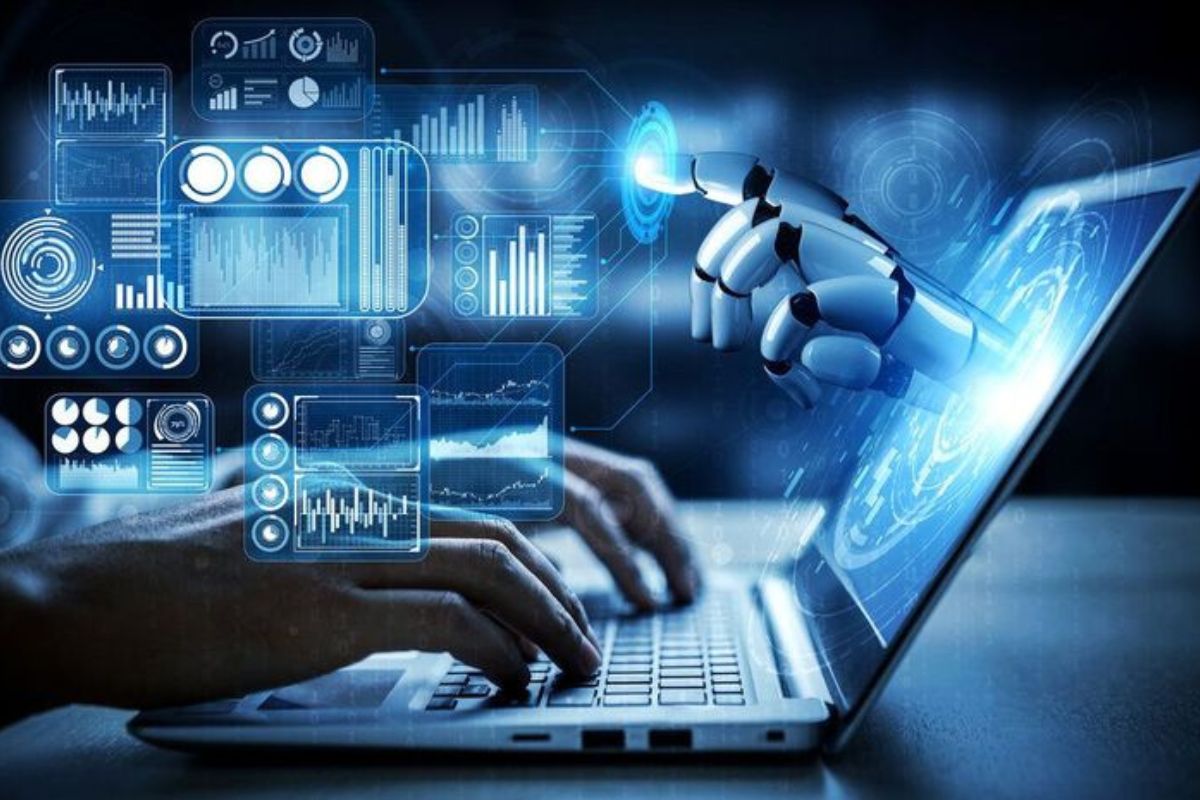
In the vast realm of human understanding, certain concepts elude easy definition or classification. One such term that has recently garnered interest and curiosity is possiblyethereal. This intriguing term blends the boundaries of the tangible and the intangible, inviting us to explore the mysterious dimensions that lie beyond our everyday perception. In this article, we will delve deep into the meaning and implications of possiblyethereal, its relevance in various fields, and its potential impact on our understanding of the universe.
Defining Possiblyethereal
The term “possiblyethereal” is a portmanteau of “possible” and “ethereal.” The word “ethereal” is often associated with something delicate, light, and otherworldly, transcending the mundane physical realm. When combined with “possible,” it suggests a concept that exists on the brink of realization, hovering between reality and imagination. Possiblyethereal thus refers to phenomena or entities that might exist in an ethereal form, not entirely bound by physical laws but still within the realm of possibility.
The Origins of Possiblyethereal
The concept of possiblyethereal has roots in various philosophical and metaphysical traditions. Ancient cultures often spoke of realms and beings that existed beyond the material world. In Greek mythology, the gods and goddesses were believed to reside in an ethereal plane, influencing the mortal world from a distance. Similarly, many Eastern philosophies propose the existence of subtle energies and dimensions that are not perceptible through ordinary senses.
In modern times, possible ethereal has found resonance in discussions about quantum physics, consciousness, and the nature of reality. The term has been adopted by thinkers and researchers who are exploring the fringes of scientific understanding, where conventional explanations often fall short.
Possiblyethereal in Science and Technology
The exploration of possible ethereal phenomena has significant implications for science and technology. In quantum physics, for instance, particles exhibit behaviors that challenge our traditional notions of reality. Quantum entanglement and superposition suggest that particles can exist in multiple states simultaneously, a concept that aligns with the idea of possiblyethereal entities.
Moreover, advancements in technology are pushing the boundaries of what we consider possible. Virtual reality (VR) and augmented reality (AR) create immersive experiences that blend the digital and physical worlds, giving us a glimpse into possiblyethereal environments. These technologies allow us to interact with digital constructs that feel real, yet exist in an intangible form.
Possiblyethereal in Art and Literature
Art and literature have long been mediums through which humans explore the ethereal and the possible. The concept of possiblyethereal finds expression in various artistic and literary works that challenge our perceptions and invite us to ponder the unknown.
In literature, possiblyethereal themes are evident in genres such as fantasy and science fiction. Authors create worlds and characters that exist in a liminal space, where the boundaries between reality and imagination blur. These narratives often explore the implications of possiblyethereal beings and phenomena on human life and society.
Artists, too, have been inspired by the idea of possiblyethereal. Visual art, in particular, can convey the delicate, otherworldly quality of ethereal subjects. Abstract and surrealist art movements have produced works that evoke a sense of the possiblyethereal, inviting viewers to engage with the unknown.
The Philosophical Implications of Possiblyethereal
Possiblyethereal concepts challenge our understanding of reality and existence. Philosophers have long grappled with questions about the nature of being and the limits of human knowledge. The idea of possiblyethereal invites us to consider that reality might be more complex and layered than we can perceive.
One philosophical perspective that resonates with possible ethereal is the notion of panpsychism, which posits that consciousness is a fundamental aspect of all matter. This idea suggests that even inanimate objects might possess a form of consciousness, existing in a possible ethereal state. Such perspectives expand our understanding of consciousness and its relationship to the material world.
Possiblyethereal and Spirituality
The possiblyethereal concept also intersects with spirituality and metaphysical beliefs. Many spiritual traditions propose the existence of higher planes of reality and beings that inhabit them. These ethereal realms are often seen as sources of wisdom, guidance, and healing.
Meditation and other spiritual practices are sometimes described as methods for accessing possiblyethereal dimensions. Practitioners report experiences of transcending ordinary reality and connecting with subtle energies or entities. These experiences can provide insights and transformative effects, suggesting that the possible ethereal has practical implications for personal growth and well-being.
Possiblyethereal in Modern Culture
In contemporary culture, the fascination with possiblyethereal is evident in various forms of media and entertainment. Films, television shows, and video games frequently explore themes of alternate realities, supernatural phenomena, and otherworldly beings.
The popularity of genres like fantasy and science fiction reflects a collective curiosity about the possiblyethereal. These stories allow audiences to engage with ideas that challenge the limits of known reality, sparking imagination and wonder.
The Future of Possiblyethereal Exploration
As our understanding of the universe continues to evolve, the exploration of possiblyethereal concepts is likely to expand. Advances in science, technology, and philosophy will continue to push the boundaries of what we consider possible, revealing new dimensions of reality.
In the future, possiblyethereal research could lead to groundbreaking discoveries about the nature of consciousness, the fabric of space-time, and the interconnectedness of all things. Such discoveries could have profound implications for various fields, from physics to psychology, and could transform our understanding of the universe and our place within it.
Conclusion
The concept of possiblyethereal invites us to explore the edges of reality and imagination, challenging our perceptions and expanding our horizons. Whether through science, art, philosophy, or spirituality, the possiblyethereal offers a rich and multifaceted lens through which to view the world. As we continue to investigate these elusive phenomena, we may uncover deeper truths about the nature of existence and the mysteries that lie beyond.
FAQs
1. What does the term possiblyethereal mean?
Possiblyethereal refers to phenomena or entities that exist on the brink of realization, hovering between reality and imagination. It suggests a concept that is not entirely bound by physical laws but still within the realm of possibility.
2. How does possiblyethereal relate to quantum physics?
In quantum physics, particles exhibit behaviors that challenge traditional notions of reality, such as quantum entanglement and superposition. These concepts align with the idea of possiblyethereal entities, which exist in multiple states simultaneously.
3. Can possiblyethereal phenomena be experienced through technology?
Yes, advancements in technology, such as virtual reality (VR) and augmented reality (AR), create immersive experiences that blend the digital and physical worlds. These technologies allow us to interact with digital constructs that feel real, yet exist in an intangible form.
4. How is possiblyethereal explored in art and literature?
Possiblyethereal themes are evident in genres such as fantasy and science fiction, where authors create worlds and characters that exist in a liminal space. Visual art, particularly abstract and surrealist movements, also evokes the delicate, otherworldly quality of ethereal subjects.
5. What are the philosophical implications of possiblyethereal?
Possiblyethereal concepts challenge our understanding of reality and existence. Philosophical perspectives such as panpsychism, which posits that consciousness is a fundamental aspect of all matter, suggest that reality might be more complex and layered than we can perceive.
You may like
Blog
Orderkens.com: Your Ultimate Destination for Unmatched Online Shopping
Published
3 days agoon
September 14, 2024By
Rock seo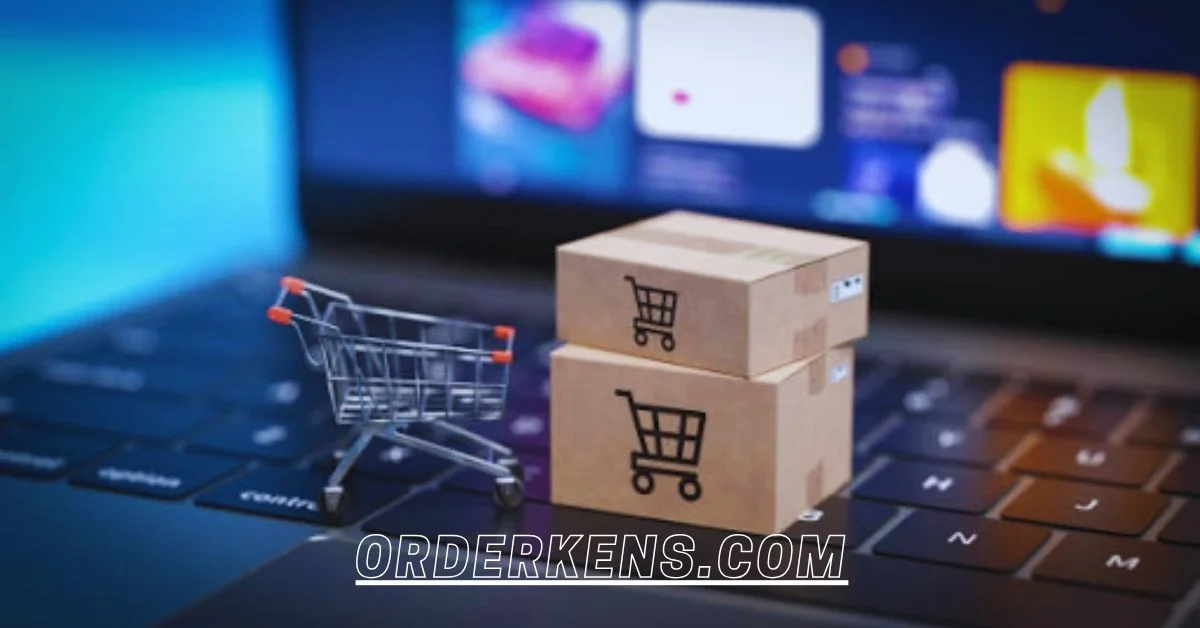
In today’s fast-paced world, convenience is paramount, and online shopping has become a preferred method for millions globally. Whether you’re looking for the latest tech gadgets, home essentials, or fashion-forward clothing, a reliable platform makes all the difference. Orderkens.com, a one-stop destination, has risen to prominence as a go-to platform, catering to all your shopping needs while providing unbeatable deals, a wide product range, and exceptional customer service.
Why Orderkens.com Stands Out in Online Shopping
Online shopping sites are a dime a dozen. What sets Orderkens.com apart from the rest is its commitment to providing an excellent customer experience, diverse product selection, and competitive prices. Whether you’re a frequent online shopper or someone who occasionally browses, the platform is designed to meet various needs, all while ensuring ease of use and satisfaction at every step.
Orderkens.com offers the best of both worlds—a vast selection of products and seamless functionality. From electronics to fashion to home goods, it’s a shopper’s paradise. But there’s more to this platform than just a wide range of products. The user-friendly interface, timely shipping, and excellent after-sales service make it a top choice for shoppers who value convenience and reliability.
Exploring the Product Range at Orderkens.com
At Orderkens.com, the product variety is nothing short of astounding. Whether you’re hunting for the latest trends in fashion, tech gadgets, home and kitchen appliances, or beauty and skincare products, this site has it all. The store sections are carefully organized to allow you to find what you’re looking for quickly and efficiently.
The fashion section offers a comprehensive range of clothing and accessories for men, women, and children. Meanwhile, the electronics section is a tech lover’s dream, featuring everything from smartphones and laptops to smart home devices. Homeowners will also appreciate the vast array of household products, from furniture and décor to kitchen essentials.
Unbeatable Deals and Offers: What Keeps Shoppers Coming Back to Orderkens.com
One of the major draws of Orderkens.com is its exclusive deals and offers, which cater to budget-conscious shoppers without compromising quality. The platform frequently updates its promotions, including flash sales, seasonal discounts, and loyalty rewards, ensuring customers always have access to affordable options.
It’s also common to find bundle deals that allow customers to buy multiple items at a reduced price, and discounts that apply to specific categories like tech, fashion, or home goods. Many regular shoppers swear by the platform’s ability to deliver high-quality products at the best prices, making it a cost-effective solution for all their shopping needs.
User-Friendly Website and Shopping Experience
When it comes to ease of use, Orderkens.com is designed with the customer in mind. The website is intuitively laid out, with clear categories and sub-categories to ensure that users can navigate effortlessly. Searching for products is simplified with robust filters that allow users to sort items by price, popularity, and relevance.
Additionally, the checkout process is seamless and secure, offering multiple payment methods, including credit cards, PayPal, and even installment plans for larger purchases. The site’s mobile-friendly design ensures that whether you’re shopping from a desktop or a smartphone, your experience is hassle-free.
Orderkens.com: A Platform Built on Customer Satisfaction
A key factor that has driven Orderkens.com to become a household name is its unwavering commitment to customer satisfaction. From responsive customer support to flexible return policies, the platform puts customer needs first. The team behind Orderkens.com understands that shopping online comes with its uncertainties, which is why they’ve instituted a clear and simple returns policy that gives customers peace of mind.
The site also provides comprehensive customer reviews on each product, helping shoppers make informed decisions. If you’re not sure about a product, you can easily browse through the ratings and reviews left by other customers, which adds another layer of trust and reliability to the shopping process.
Speedy Delivery and Tracking Options at Orderkens.com
Delivery speed is another area where Orderkens.com excels. For those who can’t wait to get their hands on their purchases, the site offers fast shipping options on most products. Whether you’re ordering a gift at the last minute or simply eager to start using your new gadget, the expedited delivery choices make shopping all the more enjoyable.
Additionally, Orderkens.com provides easy-to-use tracking tools, allowing you to monitor your order’s progress from the warehouse to your doorstep. With regular updates and transparent shipping policies, you’ll never have to wonder where your package is or when it will arrive.
Orderkens.com’s Commitment to Sustainable Practices
As consumers become more conscious of their environmental impact, Orderkens.com has taken steps to integrate sustainable practices into its operations. The platform offers a range of eco-friendly products, including sustainable fashion items, organic skincare products, and energy-efficient appliances.
Moreover, the company has reduced its carbon footprint by implementing green packaging solutions and offering carbon-neutral delivery options. This commitment to sustainability resonates with eco-conscious shoppers and shows that Orderkens.com is more than just a place to shop—it’s a platform that cares about the future of the planet.
Orderkens.com’s Loyalty Program and Membership Perks
Frequent shoppers will be pleased to know that Orderkens.com offers a loyalty program that rewards customers for their continued patronage. Members can earn points for every purchase, which can then be redeemed for discounts on future orders. The platform also offers exclusive early access to sales, special promotions, and free shipping for members, making it a worthwhile investment for regular buyers.
Membership perks go beyond just monetary savings. Members often receive personalized recommendations based on their shopping history, making it easier to discover new products that align with their tastes and preferences.
Why You Should Choose Orderkens.com for Your Next Purchase
In a world where online shopping options are endless, Orderkens.com has set itself apart as a trustworthy and customer-focused platform. Its wide range of products, competitive pricing, and dedication to customer satisfaction make it the ultimate destination for savvy shoppers. Whether you’re in search of the latest fashion trends, must-have tech gadgets, or sustainable household items, Orderkens.com has everything you need—and more.
FAQs
What types of products can I find on Orderkens.com? Orderkens.com offers a vast range of products, including fashion, electronics, home and kitchen goods, beauty products, and more.
Does Orderkens.com offer discounts or special deals? Yes, Orderkens.com regularly features exclusive deals, flash sales, and seasonal promotions that allow customers to save on various products.
How fast is the delivery from Orderkens.com? Orderkens.com offers multiple shipping options, including expedited delivery for those who need their orders quickly. You can track your orders easily with their tracking tool.
What payment methods does Orderkens.com accept? Orderkens.com accepts various payment options, including credit cards, PayPal, and installment payment plans for larger purchases.
Is there a return policy on Orderkens.com? Yes, Orderkens.com offers a flexible return policy, allowing customers to return or exchange items within a certain period, provided they meet the return conditions.
Does Orderkens.com have a loyalty program? Yes, Orderkens.com has a loyalty program where customers can earn points for every purchase and redeem them for discounts on future orders. Members also receive additional perks like free shipping and early access to sales.
Conclusion
Orderkens.com has proven itself to be more than just another online store—it’s a complete shopping experience tailored to meet modern consumers’ needs. With a vast array of products, attractive deals, and a user-friendly platform, it’s clear why so many people turn to Orderkens.com for their shopping needs. Furthermore, the platform’s commitment to sustainability and customer satisfaction ensures that every shopping experience is positive, rewarding, and eco-conscious.
If you’re looking for a hassle-free shopping destination that combines variety, value, and reliability, look no further than Orderkens.com—your ultimate destination for online shopping.
Blog
Exploring Clochant: The Art of Crafting Unique
Published
4 days agoon
September 13, 2024By
Rock seo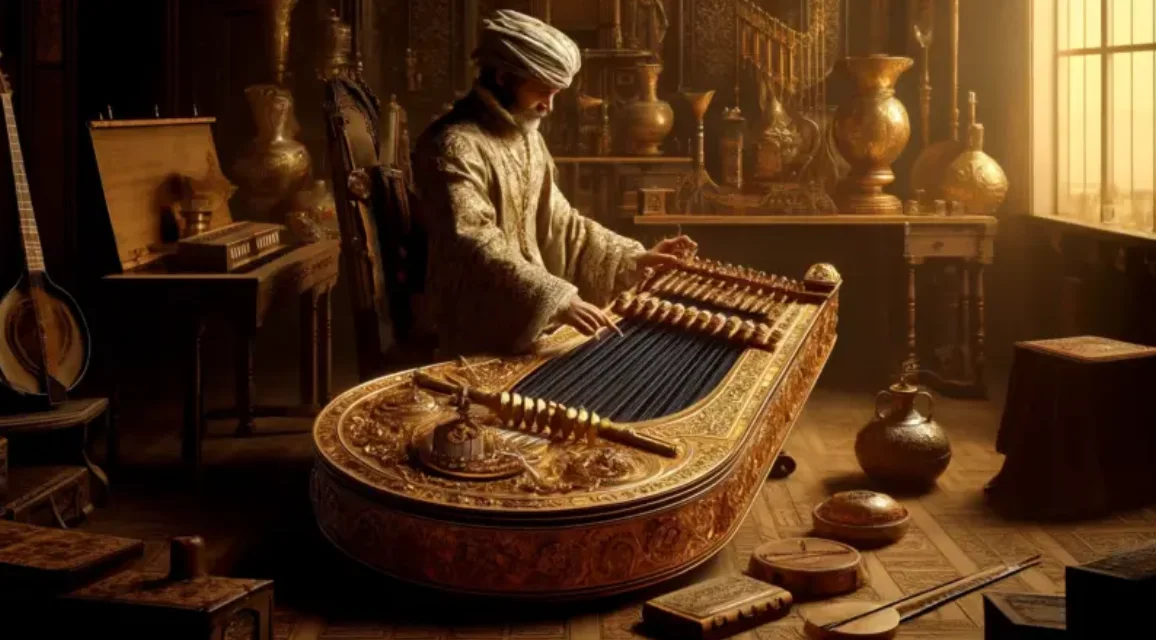
Clochant represents an artistic philosophy where creativity and craftsmanship come together to forge something truly unique. Rooted in time-honored traditions yet embracing innovative techniques, Clochant has gained recognition among connoisseurs of artisan craftsmanship. Whether you are an artist, a crafts enthusiast, or simply someone who appreciates the finer details of handcrafted work, Clochant offers a glimpse into the evolving world of bespoke artistry.
The process behind Clochant is as intricate as the creations it brings to life. Each piece is meticulously designed and crafted, focusing on individual expression and authenticity. It is not just about creating objects; it’s about telling stories, evoking emotions, and leaving a lasting impression through craftsmanship that stands the test of time.
What Makes Clochant Unique?
In a world where mass production often overshadows originality, Clochant is a breath of fresh air. At its core, Clochant emphasizes individuality. No two pieces are the same, as each work reflects the artist’s vision and skill. Whether it’s jewelry, furniture, or decorative art, the value of Clochant lies in its uniqueness. The imperfections that arise from handcrafting add to the charm, turning what might otherwise be considered flaws into integral parts of the design.
Moreover, Clochant brings together various techniques, blending old-world craftsmanship with modern aesthetics. Artists often draw inspiration from their environment, personal experiences, or cultural heritage. This fusion creates works that are not only visually striking but also deeply personal.
The Evolution of Artisan Craftsmanship
The roots of Clochant can be traced back to the early days of craftsmanship, where artisans honed their skills through years of practice and apprenticeship. However, with industrialization, much of this artisanal knowledge was at risk of being lost. Clochant emerged as a response to this shift, ensuring that traditional skills are preserved while being adapted to modern tastes.
Today, the rise of Clochant symbolizes a return to slow, intentional production. Artists are encouraged to take their time, perfect their techniques, and experiment with materials. This movement is as much about the creative process as it is about the final product. In a world that often values speed and efficiency, Clochant champions patience and dedication.
Techniques and Materials in Clochant Crafting
Clochant artisans employ a wide range of materials and techniques, depending on their specialty. From intricate woodworking to delicate metal forging, each craft requires a different set of skills and tools. Let’s take a closer look at some of the most common methods used in Clochant:
- Woodworking: Artists might use traditional carving techniques to bring a raw piece of wood to life, creating furniture or decorative sculptures. The wood’s grain and natural imperfections become part of the finished piece, making each item one of a kind.
- Metalworking: Clochant metalworkers often create jewelry or ornamental pieces, using age-old forging methods. Whether working with gold, silver, or more industrial metals, each piece is meticulously shaped by hand, giving it a unique form.
- Textile Arts: In Clochant, textiles are not just functional but also a canvas for creativity. Artisans might use hand-dyeing techniques, weaving, or embroidery to create stunning garments or fabric art. Every stitch and thread tells a story.
- Ceramics: Pottery is another important facet of Clochant. Unlike mass-produced ceramics, each Clochant piece is formed and painted by hand, with no two pots or dishes looking exactly alike. Glazing techniques, texture, and form are unique to the artist’s vision.
The Role of Sustainability in Clochant
As more consumers become aware of the environmental impact of mass production, Clochant has positioned itself as a sustainable alternative. Artisans prioritize sourcing materials responsibly, often using recycled or locally sourced elements. Furthermore, the emphasis on quality over quantity means that Clochant pieces are made to last, reducing the need for frequent replacements.
This slow fashion and crafting movement also encourages consumers to think more carefully about the items they purchase. When you invest in a piece of Clochant art, you are supporting a sustainable, ethical approach to production that values artistry and the environment equally.
Finding Your Unique Clochant Piece
The beauty of Clochant lies in its diversity. Whether you are searching for a statement piece of furniture, a unique piece of jewelry, or a handcrafted garment, there is something for every taste. Many artists offer custom commissions, allowing you to collaborate on a piece that reflects your personal style or story.
Clochant galleries and artisan markets are some of the best places to discover these unique creations. Here, you can meet the artists, learn about their creative process, and find items that resonate with you on a deeper level. The personal connection to the artist often adds another layer of meaning to the piece, making it even more special.
FAQs
What is Clochant, and how does it differ from other artisanal crafts?
Clochant emphasizes the individuality of each handcrafted piece, blending traditional techniques with modern aesthetics to create truly unique works of art. It differs from other artisanal crafts by prioritizing creativity and personal expression over replication.
What materials are commonly used in Clochant crafting?
Clochant artisans work with a wide variety of materials, including wood, metals, textiles, and ceramics. Each material is chosen based on the artist’s vision, often using sustainable and locally sourced resources.
How does Clochant support sustainability?
Clochant embraces a slow production model, focusing on quality over quantity. Artisans often use recycled or ethically sourced materials, ensuring that their creations have minimal environmental impact.
Where can I find authentic Clochant pieces?
Clochant pieces can be found at artisan markets, galleries, or online platforms that specialize in handmade goods. Some artists also offer custom commissions, allowing you to personalize your purchase.
What are some popular Clochant techniques?
Popular techniques in Clochant include woodworking, metal forging, textile arts, and ceramics. Each technique requires a high level of skill and allows for creative experimentation.
How can I get involved in the Clochant movement?
To get involved, you can support local artisans by purchasing their work, attending workshops, or even trying your hand at crafting by taking courses in specific techniques like pottery, weaving, or metalworking.
Conclusion
Clochant is more than just an art form; it’s a movement that values individuality, creativity, and sustainability. In a world driven by mass production and uniformity, Clochant stands as a testament to the power of handmade, unique craftsmanship. Whether you’re an artist, a collector, or simply someone who appreciates the beauty of artisanal work, Clochant offers a world of possibilities.
By supporting this art form, you are not only investing in a one-of-a-kind piece but also contributing to the preservation of traditional craftsmanship in a modern context.
Blog
Easy Job Search Tools & Resources on Jobzvice.com
Published
1 week agoon
September 9, 2024By
Rock seo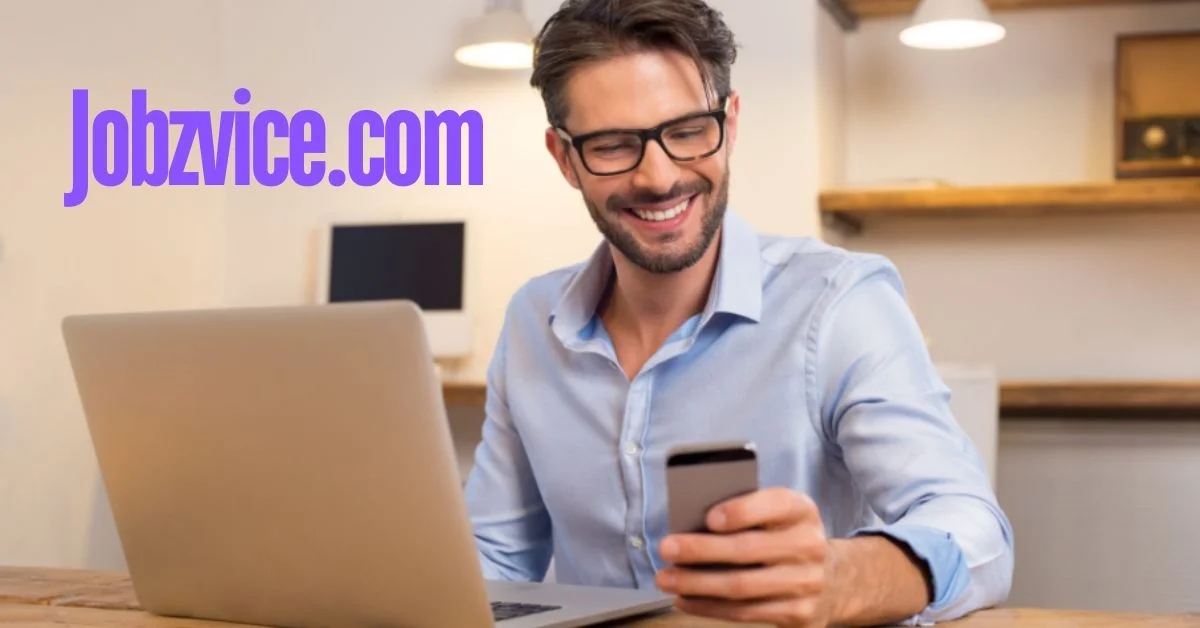
In today’s fast-paced world, finding the right job can be a daunting task. With countless platforms offering similar services, it’s easy to get lost in the shuffle. Enter Jobzvice.com, a platform designed to make job hunting more efficient and less stressful. By offering a suite of easy-to-use tools and valuable resources, Jobzvice.com has carved out a space for itself in the competitive job search landscape. But what makes this platform stand out, and how can it help you find the perfect job? Let’s dive into the features and resources offered by Jobzvice.com and how they simplify the entire process.
Jobzvice.com: Simplifying the Job Hunt
When you’re on the lookout for a new career opportunity, navigating multiple platforms, researching companies, and refining resumes can feel overwhelming. Jobzvice.com understands these struggles and aims to streamline the process by providing easy-to-use tools and relevant job search resources. From job search filters to resume building, the platform offers a one-stop solution for job seekers.
One of the key features is its clean, user-friendly interface. Unlike other job portals that bombard users with endless information, Jobzvice.com provides a simplified browsing experience, making it easier to focus on what truly matters — landing your dream job.
Efficient Search Tools
Jobzvice.com prides itself on offering efficient search tools that allow you to narrow down your search based on industry, location, job type, and experience level. Instead of scrolling through pages of irrelevant listings, the site helps you pinpoint the roles that best suit your skills and preferences.
The advanced search filter ensures that your results are tailored to your unique career needs. Whether you’re looking for full-time, part-time, freelance, or remote opportunities, Jobzvice.com covers a broad spectrum of job types, making it easier to find a job that fits your lifestyle.
Job Alerts for Real-Time Updates
Keeping track of job postings can be tricky. Often, you may miss out on opportunities simply because you didn’t check a platform in time. With Jobzvice.com, you no longer need to worry about missing out. The Job Alert feature keeps you informed by sending real-time notifications about new job postings that match your search criteria.
This tool enables you to stay ahead of the curve and apply for roles as soon as they become available, giving you a competitive edge in the job market.
Job Application Tracker
One of the challenges job seekers often face is keeping track of all their applications. Jobzvice.com eliminates this hassle with its job application tracker. This tool helps you organize all your job applications in one place, so you can easily monitor the status of each one.
No more juggling multiple tabs or struggling to remember which jobs you’ve applied to — the tracker ensures you stay on top of your applications without the stress of managing them manually.
Resume and Cover Letter Resources
Your resume and cover letter are often the first things employers see, and making a good impression is crucial. Jobzvice.com offers an array of resume and cover letter resources to help you craft documents that stand out from the crowd.
With templates, writing tips, and examples tailored to different industries, the platform makes it easy for job seekers to create professional resumes and cover letters that highlight their strengths. The intuitive resume builder walks you through the process step-by-step, ensuring that your documents are polished and error-free.
Interview Preparation Tips
Landing an interview is only the first step. To secure the job, you need to impress during the interview process. Jobzvice.com doesn’t leave you hanging once you’ve submitted your application. Instead, the platform provides interview preparation resources to help you ace the next stage.
From common interview questions to tips on how to dress, Jobzvice.com equips you with everything you need to walk into your interview with confidence. You can also find advice on handling virtual interviews, which have become increasingly common in today’s job market.
Industry-Specific Job Listings
One of the standout features of Jobzvice.com is its focus on industry-specific job listings. Many job seekers are looking for roles in specialized fields, and having access to listings tailored to those industries can save time and effort.
Whether you’re in tech, healthcare, marketing, finance, or any other sector, the platform’s industry-specific filters make it easy to find positions that align with your qualifications and interests.
Company Reviews and Insights
It’s not just about finding a job — it’s about finding the right job. Jobzvice.com helps job seekers gain deeper insights into potential employers through its company review feature. Before applying, you can read reviews from current and former employees to get a sense of the company culture, work-life balance, and management style.
This transparency empowers job seekers to make more informed decisions, helping them choose companies that match their values and career goals.
Job Search Resources for Every Stage of Your Career
Whether you’re just starting your career, transitioning into a new field, or looking for a senior position, Jobzvice.com provides tailored resources for every stage. The platform offers career advice articles, tutorials, and downloadable guides that cover everything from networking tips to how to negotiate your salary.
For those just entering the job market, there are specific tools designed to help you build your first resume and cover letter. For more seasoned professionals, Jobzvice.com offers advice on advancing in your career and navigating mid-level to senior-level job searches.
Global Opportunities and Remote Work Options
As more companies adopt remote work models, job seekers are no longer confined to opportunities in their local area. Jobzvice.com recognizes this trend and offers a wide selection of remote job listings. Whether you’re looking for a job halfway across the globe or prefer the flexibility of working from home, Jobzvice.com connects you to a world of possibilities.
Additionally, the platform provides resources for navigating remote work, including tips on how to stay productive and manage time effectively when working from home.
Jobzvice.com: A Tool for Both Employers and Job Seekers
Jobzvice.com isn’t just a valuable resource for job seekers. Employers can also benefit from the platform’s range of tools to find the right candidates. By offering employer-specific features like easy job posting, applicant tracking, and candidate filtering, the platform simplifies the hiring process.
This dual functionality makes Jobzvice.com an attractive option for both sides of the job market, creating a space where talented candidates and top employers can connect efficiently.
Personalized Career Advice
Jobzvice.com goes beyond job listings to offer personalized career advice. The platform includes resources to help you identify your strengths, set career goals, and build a strategy for long-term success. By offering personalized career assessments, it helps users understand their unique skills and how to leverage them in the job market.
Whether you need help finding the right career path or advice on navigating a challenging job search, the personalized tools available on Jobzvice.com are designed to provide actionable insights that help you succeed.
FAQs
How can I sign up for job alerts on Jobzvice.com?
Signing up for job alerts is simple. You can create an account on Jobzvice.com and set your preferences based on location, industry, and job type. The platform will then notify you via email whenever a job matching your criteria is posted.
Does Jobzvice.com offer tools for remote job searches?
Yes, Jobzvice.com offers a range of remote job listings across various industries. You can filter your search to specifically target remote work opportunities, whether full-time, part-time, or freelance.
Are the resume templates on Jobzvice.com customizable?
Absolutely. Jobzvice.com provides customizable resume templates, allowing you to tailor your resume to different job applications. You can easily modify the design, layout, and content to suit your needs.
Can employers post job listings directly on Jobzvice.com?
Yes, employers can post job listings, filter candidates, and track applications all through the platform. Jobzvice.com offers tools that simplify the hiring process, making it easy for employers to find the right talent.
What industries are covered on Jobzvice.com?
Jobzvice.com covers a wide range of industries, including technology, healthcare, finance, marketing, education, and more. Whether you’re a fresh graduate or a seasoned professional, you’ll find industry-specific job listings tailored to your qualifications.
Is there a cost to use Jobzvice.com’s tools and resources?
Jobzvice.com offers a mix of free and premium tools. While many of the basic job search and application tools are free, there are advanced features and personalized resources available under a premium subscription.
Conclusion
Jobzvice.com is more than just a job search platform — it’s a comprehensive resource that supports job seekers at every stage of their career journey. From easy job search tools to personalized career advice, Jobzvice.com makes it simple to navigate the often overwhelming process of finding the right job. With features like job alerts, resume builders, and interview tips, the platform sets itself apart as a user-friendly and effective tool for job hunters worldwide.Whether you’re searching for local opportunities or aiming for a remote position, Jobzvice.com has everything you need to land your next role.
Trending
-
Lifestyle2 months ago
Geekzilla Radio: A Haven for Pop Culture Enthusiasts
-
News2 months ago
Little_mermaidd0 – A Deep Dive into Internet Identities
-
Lifestyle2 months ago
Unveiling the Mystery of rzinho: A Comprehensive Blog
-
Lifestyle2 months ago
Unveiling The Enchantment Of Şeygo: A Journey Through Turkish
-
News2 months ago
News Viko: The Ultimate Guide to Staying Informed and Connected
-
Entertainment2 months ago
Exploring the World of Валерко Логвин Архив
-
Lifestyle2 months ago
Nebraskawut Cappello: Unveiling the Mystery
-
Travel2 months ago
Mount Everest: The Sleeping Beauty in Rainbow Valley